티스토리 뷰
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0">
<display-name>tt</display-name>
<welcome-file-list>
<welcome-file>log.jsp</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>logincontroller</servlet-name>
<servlet-class>tt.login_controller</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>logincontroller</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
dbconnection.java
package database;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class dbconnect {
public static Connection getConnection()throws SQLException, ClassNotFoundException {
Connection conn = null;
String url = "jdbc:mysql://localhost:3306/tt";
String user = "root";
String password = "1234";
Class.forName("com.mysql.cj.jdbc.Driver");
conn = DriverManager.getConnection(url, user, password);
return conn;
}
}
log.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="./login.do" method="post">
<p> 아이디 : <input type="text" name="id">
<br>
<p> 비밀번호 : <input type="password" name="passwd">
<br>
<p> <input type="submit" value="전송">
</form>
</body>
</html>
log_info.java
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import database.dbconnect;
public class log_info {
private Connection dbconn;
private static log_info instance;
private log_info() {
}
public static log_info getInstance() {
if(instance == null) {
instance = new log_info();
}
return instance;
}
public String getName(String id) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
String passwd = null;
String name=null;
String sql = "select name from info where id = ? ";
try {
conn = dbconnect.getConnection();
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, id);
rs = pstmt.executeQuery();
while (rs.next()) {
name = rs.getString("name");
}
return name;
} catch (Exception ex) {
System.out.println("getname() : " + ex);
} finally {
try {
if (rs != null)
rs.close();
if (pstmt != null)
pstmt.close();
if (conn != null)
conn.close();
} catch (Exception ex) {
throw new RuntimeException(ex.getMessage());
}
}
return name;
}
public boolean check(String id, String passwd) throws ClassNotFoundException {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
String name=null;
String sql = "select * from info where id = ? and passwd=?";
try {
conn = dbconnect.getConnection();
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, id);
pstmt.setString(2, passwd);
rs = pstmt.executeQuery();
if (rs.next()) {
return true;
}
}catch(SQLException e) {
e.printStackTrace();
}
return false;
}
}
login_success.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h2>로그인 성공!</h2>
<h2><%=request.getAttribute("name") %>님 환영합니다!</h2>
<br>
<p> 사용자 아이디 : <%=request.getParameter("id") %>
<br>
<p> 사용자 비밀번호 : <%=request.getParameter("passwd") %>
<br>
<a href="./logout.do">로그아웃</a>
</body>
</html>
false.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h2>아이디, 비밀번호를 확인해주세요!</h2>
<a href="log.jsp" method="post">로그인 창으로 이동</a>
<a href="add_log.jsp" method="post">회원가입</a>
</body>
</html>
logout.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
session.invalidate();
response.sendRedirect("log.jsp");
%>
</body>
</html>
add_log.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h2>회원가입</h2>
<form action="add.do" method="post">
<p>아이디 : <input type="text" name="id">
<p>비밀번호 : <input type="text" name="passwd">
<p>이름 : <input type="text" name="name">
<p><input type="submit" value="전송">
</form>
</body>
</html>
add_info.java
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import database.dbconnect;
public class add_info {
private Connection dbconn;
private static add_info instance;
private add_info() {
}
public static add_info getInstance() {
if(instance == null) {
instance = new add_info();
}
return instance;
}
public void add(String id,String passwd,String name) throws SQLException, ClassNotFoundException {
/*
* String id = null; String passwd = null; String name = null;
*/
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
String sql = "insert into info values(?,?,?)";
try {
conn = dbconnect.getConnection();
pstmt=conn.prepareStatement(sql);
pstmt.setString(1,id);
pstmt.setString(2,passwd);
pstmt.setString(3,name);
pstmt.executeUpdate();
} catch (SQLException e) {
System.out.println("add : " + e);
}
finally {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
}
}
}
login_controller.java
package tt;
import java.io.IOException;
import java.sql.SQLException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import dao.add_info;
import dao.log_info;
public class login_controller extends HttpServlet{
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request,response);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String RequestURI = request.getRequestURI();
System.out.println("RequestURI : " +RequestURI);
String contextPath = request.getContextPath();
System.out.println("contextPath : " +contextPath);
String command = RequestURI.substring(contextPath.length());
response.setContentType("text/html P charset=utf-8");
request.setCharacterEncoding("utf-8");
if(command.equals("/login.do")) {
String id = request.getParameter("id");
String passwd = request.getParameter("passwd");
System.out.println("입력된 아이디 : "+id);
System.out.println("입력된 비밀번호 : "+passwd);
log_info info = log_info.getInstance();
boolean check = false;
try {
check = info.check(id, passwd);
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
if(check) {
String m_name = info.getName(id);
System.out.print(m_name);
request.setAttribute("name",m_name);
RequestDispatcher rd = request.getRequestDispatcher("login_success.jsp");
rd.forward(request,response);
}else {
RequestDispatcher rd = request.getRequestDispatcher("false.jsp");
rd.forward(request, response);
}
}
else if(command.equals("/logout.do")) {
RequestDispatcher rd = request.getRequestDispatcher("logout.jsp");
rd.forward(request, response);
}
else if(command.equals("/add.do")) {
String id = request.getParameter("id");
System.out.println("가입 아이디 : " +id);
String passwd = request.getParameter("passwd");
System.out.println("가입 비번 : " +passwd);
String name = request.getParameter("name");
System.out.println("가입자 이름 : " +name);
add_info dao = add_info.getInstance();
System.out.println("add 호출");
try {
dao.add(id,passwd,name);
} catch (SQLException | ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
RequestDispatcher rd = request.getRequestDispatcher("login_success.jsp");
rd.forward(request, response);
}
}
}
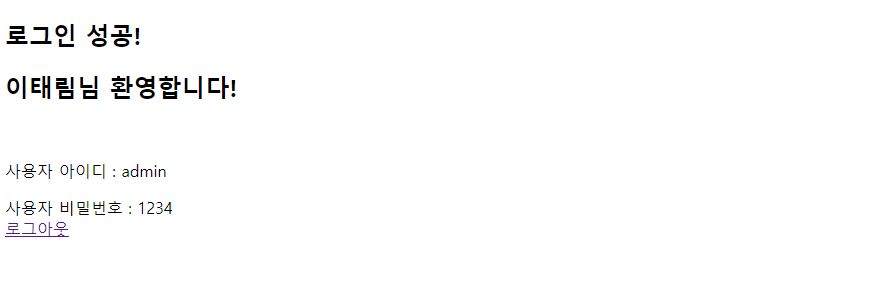

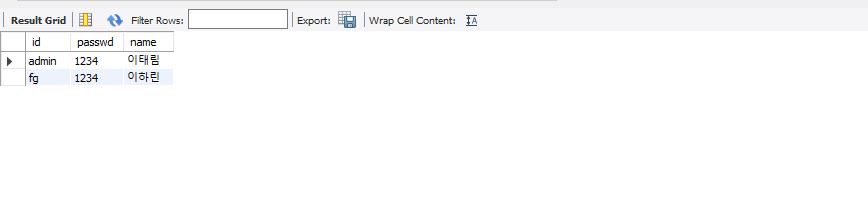
'코딩 > JSP' 카테고리의 다른 글
[12주 5일차] SendRedirect & forward (0) | 2023.12.29 |
---|---|
미니 프로젝트 (2) (0) | 2023.12.29 |
[12주 3일차] 웹 쇼핑몰 분석 (1) | 2023.12.27 |
URI / contextPath / substring (0) | 2023.12.27 |
[쉽게 배우는 JSP 웹 프로그래밍 연습문제] 18장 (0) | 2023.12.27 |
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday